Recently, I wrote an automated check-in script and found that AES encryption was used during login. I checked the JavaScript code and found it simple, so I casually wrote a Python script and recorded it briefly.
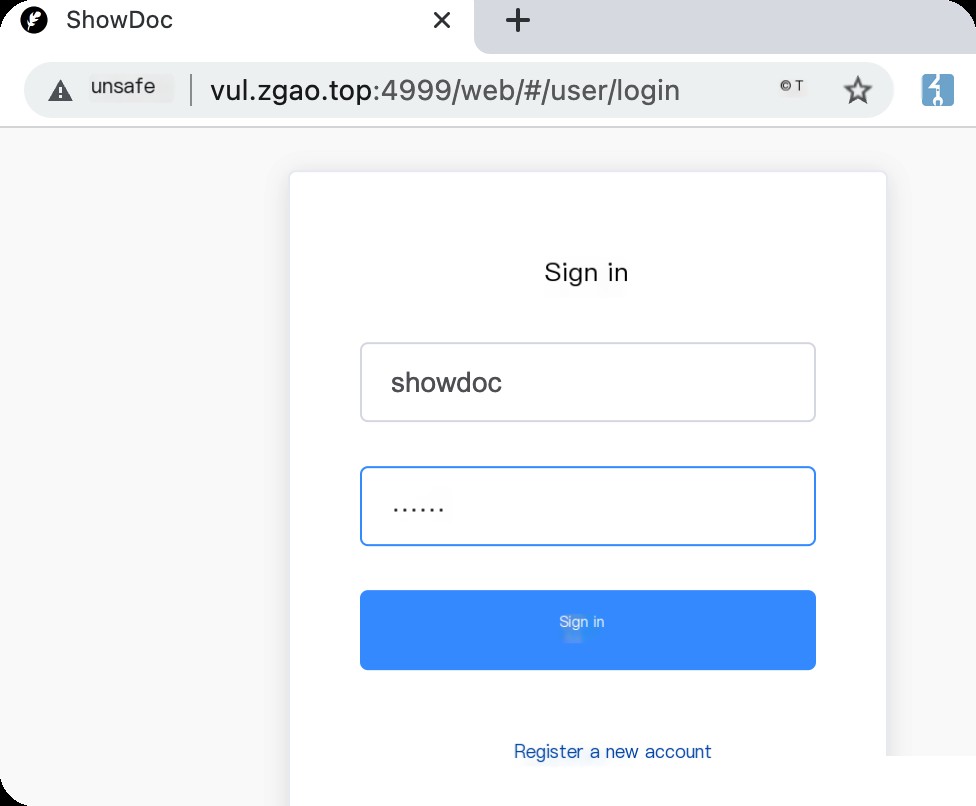
Before clicking login, the password length is normal. The image below shows the password length after clicking login. Many people might have encountered this scenario.
I guessed that the frontend processes the password before transmitting it to the backend for verification. I used Charles to capture the login parameters.
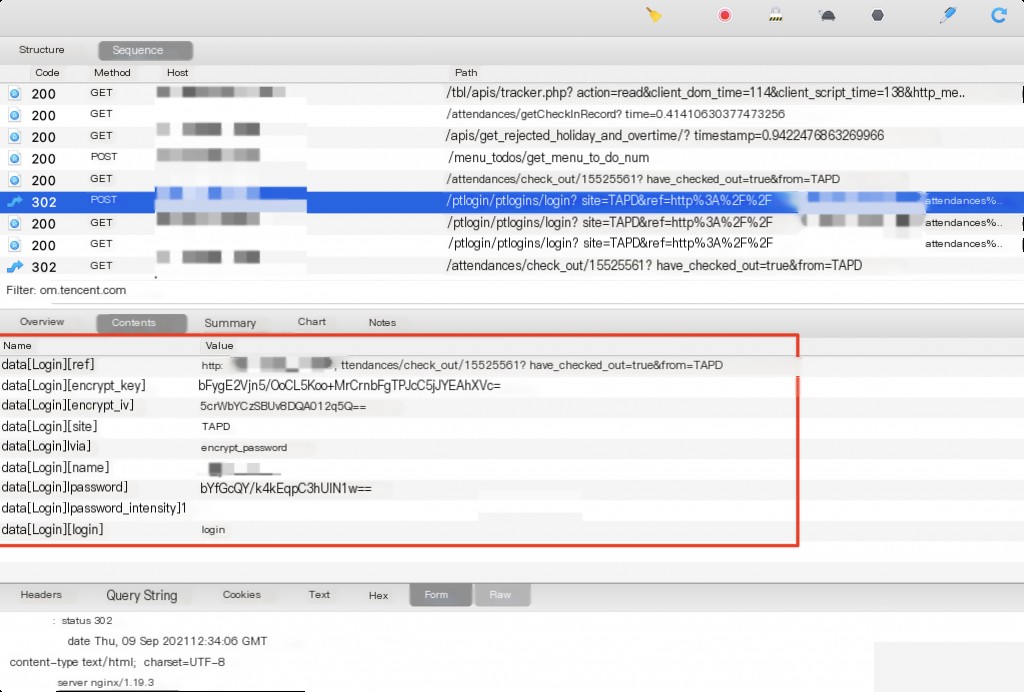
From the parameter names, it can be seen that encryption is used, and the IV offset address suggests that AES encryption is likely used. Next, we need to determine how these parameters are generated.
Open the console to check the event bound to the login button submit, quickly locating the form submission JavaScript code.
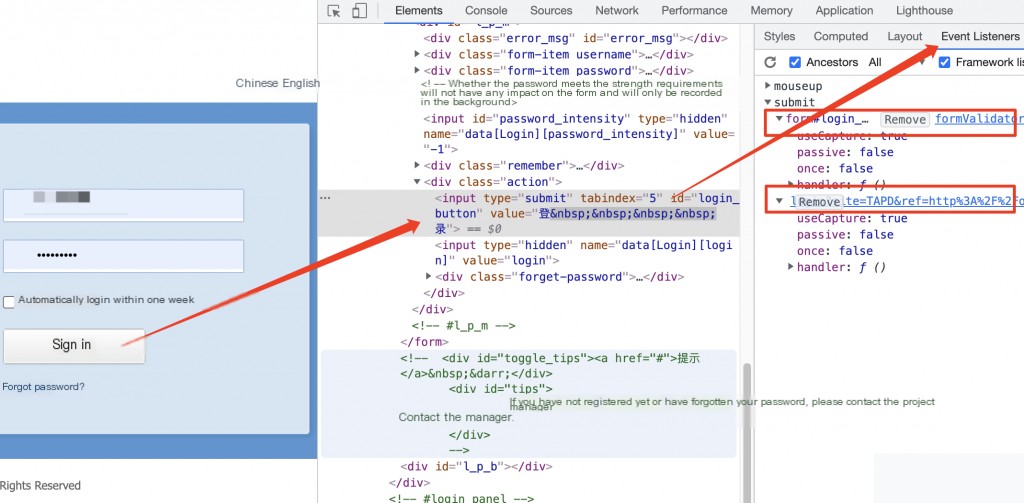
Here, two events are bound to submit. Let’s check the corresponding code for each.
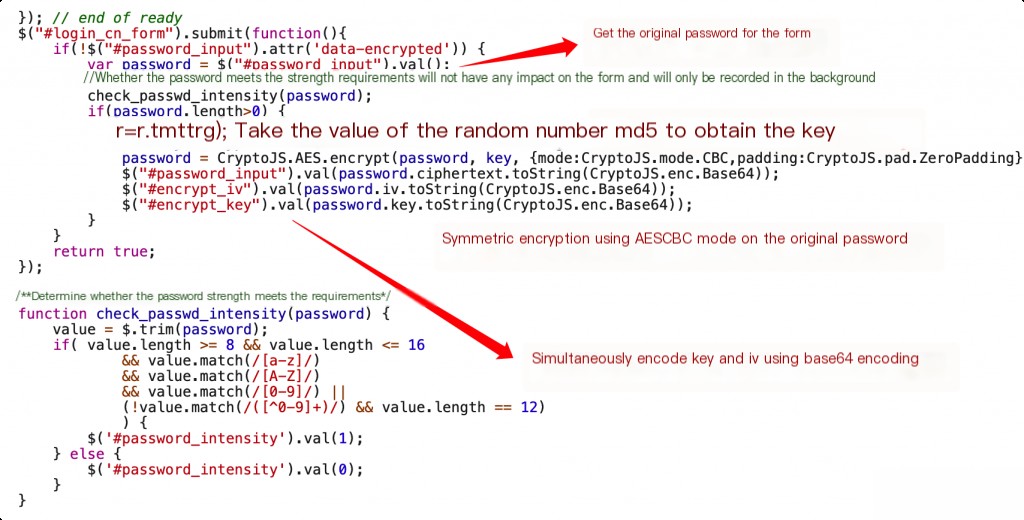
Comparing the two bound JavaScript events, they are found to be roughly the same.
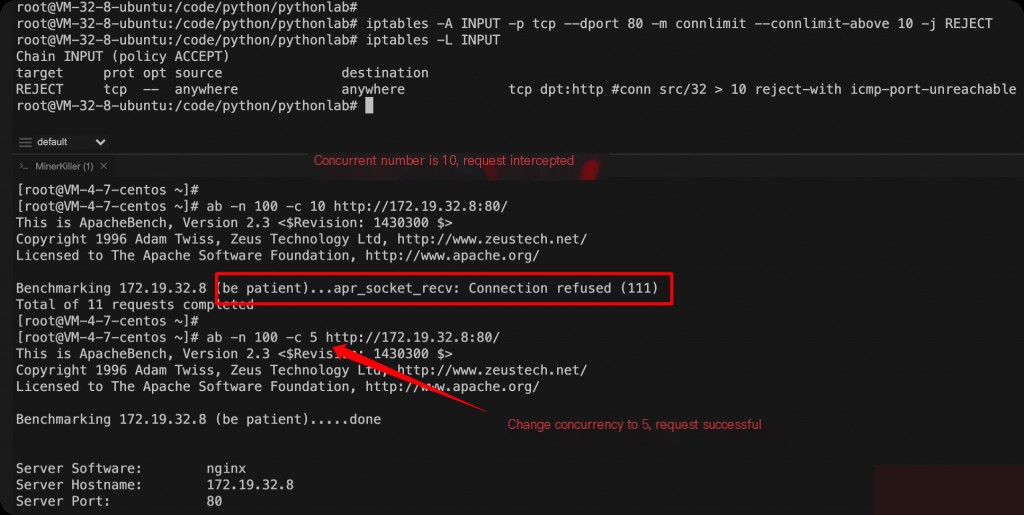
Having understood the encryption logic, I started debugging the JavaScript.
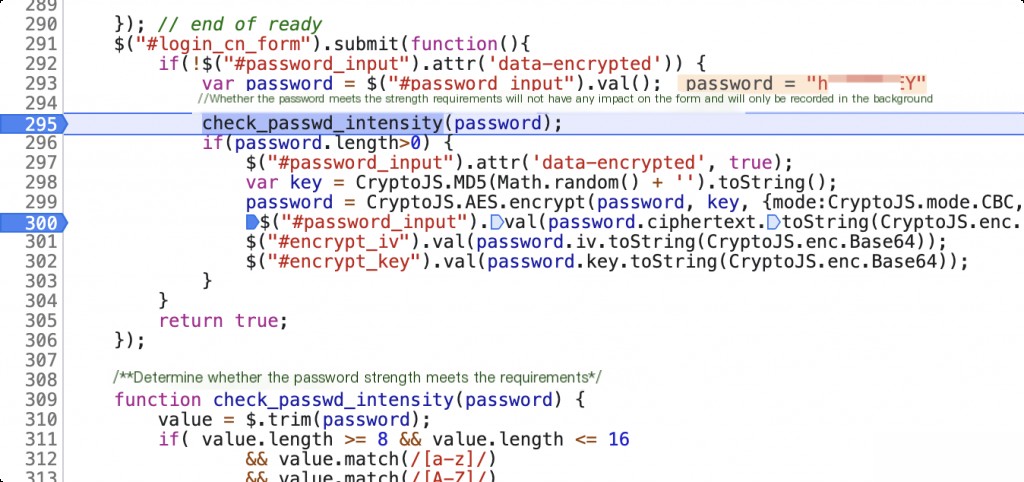
Set a breakpoint at line 295 to check the password complexity. At this point, the password is the original password, as in the initial state of the form above.
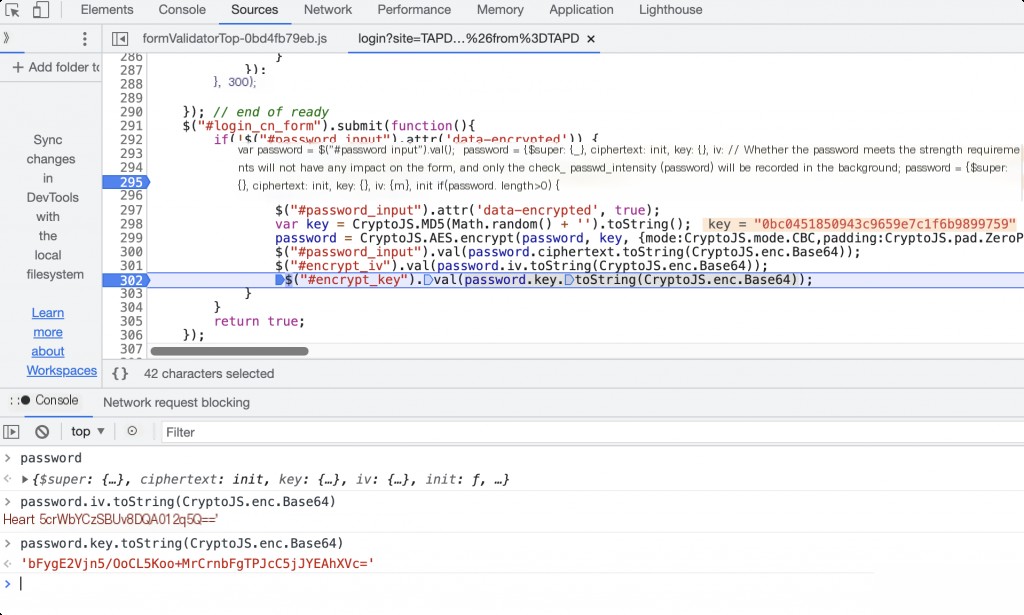
Step through the code, and you can see each encrypted value.
It turns out to be quite simple. After analyzing the login logic, I can start writing the script.
How can this JavaScript encryption logic be implemented in Python code? I thought of two approaches:
- Directly extract the JavaScript code, use the execjs library to execute the JavaScript code and get the encryption result
- Rewrite the JavaScript code in Python
Both approaches are feasible since they are not complex.
How to extract JavaScript code for execution?
import execjs jsCode = ''' var CryptoJS = require("crypto-js"); function jsAES(){ var password = "xxxxx"; var key = CryptoJS.MD5(Math.random() + '').toString(); password = CryptoJS.AES.encrypt(password, key, {mode:CryptoJS.mode.CBC,padding:CryptoJS.pad.ZeroPadding}); var newPassword = password.ciphertext.toString(CryptoJS.enc.Base64); var iv = password.iv.toString(CryptoJS.enc.Base64); var key = password.key.toString(CryptoJS.enc.Base64); return [newPassword,iv,key] } ''' ctx = execjs.compile(jsCode) password, iv, key = ctx.call("jsAES") print(password,iv,key)
Since AES encryption is used, the crypto-js module needs to be required when converting to Node.js. Other parts can be copied over. To facilitate Python handling the variables after JavaScript execution, the JavaScript function can return an array.
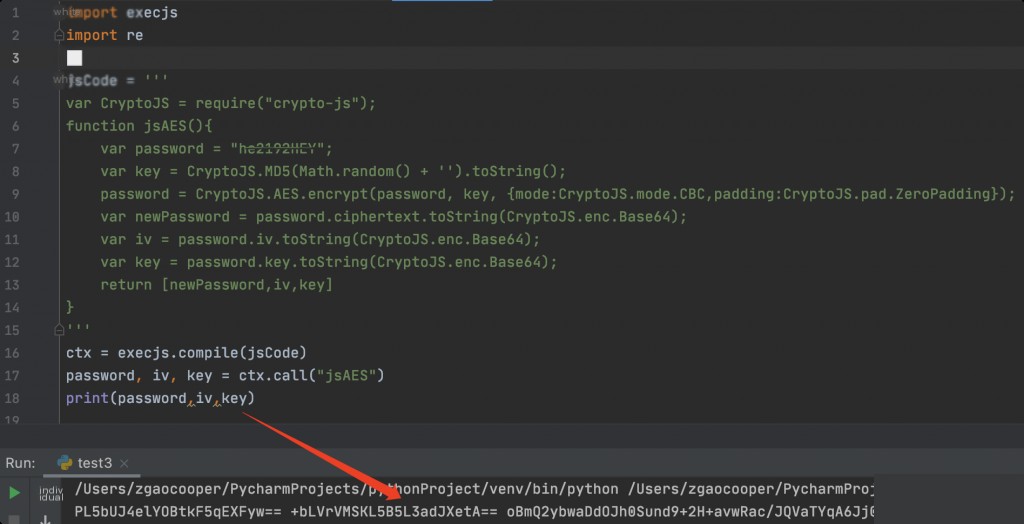
After completing the login script, the check-in part follows.
The rest is too simple to write about. A few years ago, I might have wanted to write it down, but now I only want to write the important parts.